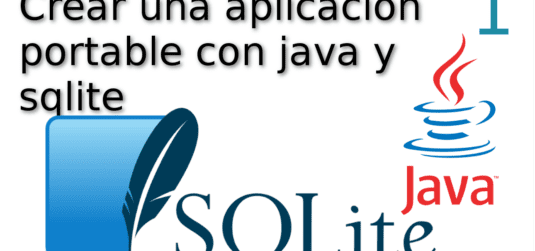
01.- Crear una aplicación portable con java y sqlite ?
pom.xml
<dependencies> <dependency> <groupId>org.xerial</groupId> <artifactId>sqlite-jdbc</artifactId> <version>3.8.11.2</version> </dependency> <dependency> <groupId>unknown.binary</groupId> <artifactId>AbsoluteLayout</artifactId> <version>SNAPSHOT</version> </dependency> </dependencies>
NewJFrame.java
package com.eucm.sqlite1; import java.sql.Connection; import java.sql.DriverManager; import java.sql.ResultSet; import java.sql.SQLException; import java.sql.Statement; import javax.swing.table.DefaultTableModel; public class NewJFrame extends javax.swing.JFrame { Connection connection = null; Statement statement = null; DefaultTableModel modelo=new DefaultTableModel(); private Connection connect() { Connection conn = null; try { conn = DriverManager.getConnection("jdbc:sqlite:database.db"); } catch (SQLException e) { System.out.println(e.getMessage()); } return conn; } public NewJFrame() { initComponents(); modelo.addColumn("id"); modelo.addColumn("nombre"); modelo.addColumn("direccion"); String Dato[]=new String[3]; modelo.setRowCount(0); try { //CONECTA A LA BD connection = this.connect(); statement = connection.createStatement(); statement.setQueryTimeout(20); //QUERY QUE JALA TODAS LAS PUBLICACIONES String query = "SELECT " + "id, " + "nombre, " + "direccion " + "FROM personal;"; ResultSet rs = statement.executeQuery(query); //CICLO QUE LLENA TODO EL MODELO while (rs.next()) { Dato[0]=rs.getString("id"); Dato[1]=rs.getString("nombre"); Dato[2]=rs.getString("direccion"); modelo.addRow(Dato); } //LLENAMOS LA TABLA CON EL MODELO tabla.setModel(modelo); //OCULTAMOS EL ID EN LA TABLA //tabla.getColumn("id").setMaxWidth(50); } catch (SQLException e) { System.err.println(e.getMessage()); } finally { try { if (connection != null) { connection.close(); } } catch (SQLException e) { // connection close failed. System.err.println(e); } } } }
database.db
PRAGMA foreign_keys = OFF; CREATE TABLE "personal" ( "id" INTEGER PRIMARY KEY AUTOINCREMENT, "nombre" TEXT, "direccion" TEXT ); INSERT INTO "main"."personal" VALUES (1, 'eugenio', 'mexico');
Para poder compilar nuestro proyecto y hacerlo portable debemos agregar este codgo a pom.xml
<!-- CODIGO A AGREGAR PARA PODER CREAR UN .JAR --> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-dependency-plugin</artifactId> <executions> <execution> <id>copy-dependencies</id> <phase>prepare-package</phase> <goals> <goal>copy-dependencies</goal> </goals> <configuration> <outputDirectory>${project.build.directory}/lib</outputDirectory> <overWriteReleases>false</overWriteReleases> <overWriteSnapshots>false</overWriteSnapshots> <overWriteIfNewer>true</overWriteIfNewer> </configuration> </execution> </executions> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-jar-plugin</artifactId> <configuration> <archive> <manifest> <addClasspath>true</addClasspath> <classpathPrefix>lib/</classpathPrefix> <mainClass>com.eucm.sqlite3.NewJFrame</mainClass> </manifest> </archive> </configuration> </plugin> </plugins> </build> <!-- FIN -->
donde
com.eucm.sqlite3.NewJFrame=Es el path virtual de nuestra clase principal