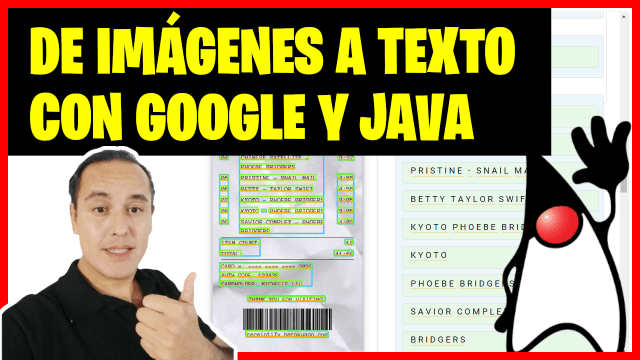
Convertir de imágenes a texto con Java (OCR de Google)
Ahora que hemos configurado el API de Google Visión vamos a Convertir de imágenes a texto con Java y usaremos como IDE a NetBeans siguiendo estos pasos:
Configuramos el proyecto en NetBeans
Creamos un nuevo proyecto de tipo Java whit Maven y hacemos click en siguiente
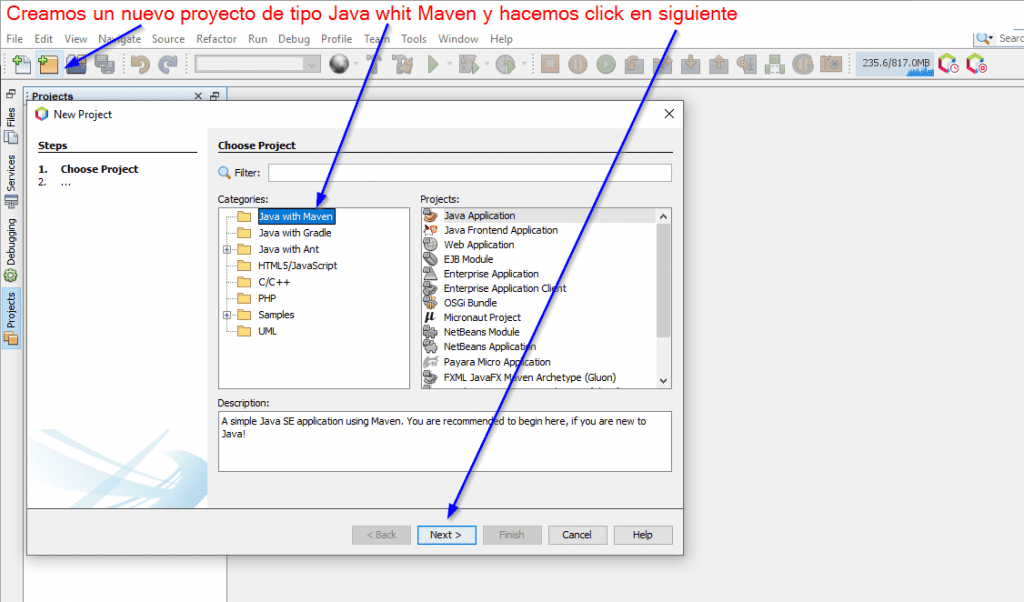
Colocamos un nombre y click en Finish
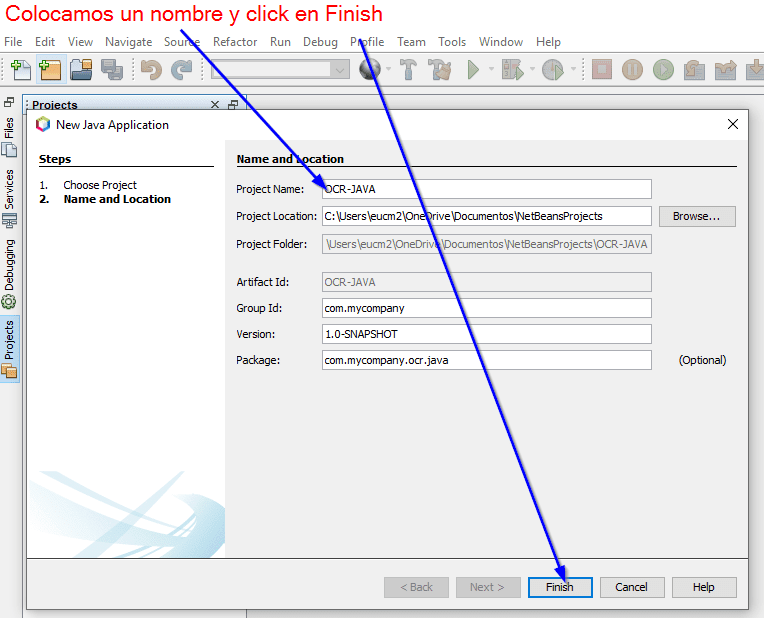
Abrimos pom.xml y desde https://cloud.google.com/vision/docs/detect-labels-image-client-libraries#client-libraries-usage-java agregamos e código
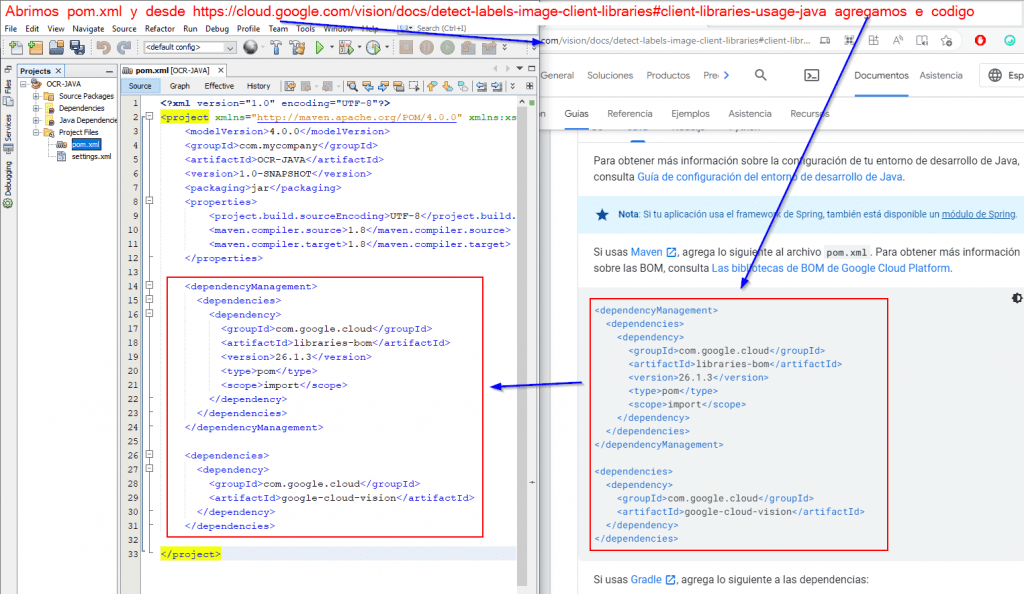
<dependencyManagement> <dependencies> <dependency> <groupId>com.google.cloud</groupId> <artifactId>libraries-bom</artifactId> <version>26.1.3</version> <type>pom</type> <scope>import</scope> </dependency> </dependencies> </dependencyManagement> <dependencies> <dependency> <groupId>com.google.cloud</groupId> <artifactId>google-cloud-vision</artifactId> </dependency> </dependencies>
Agregamos un Java Main Class en Netbeans
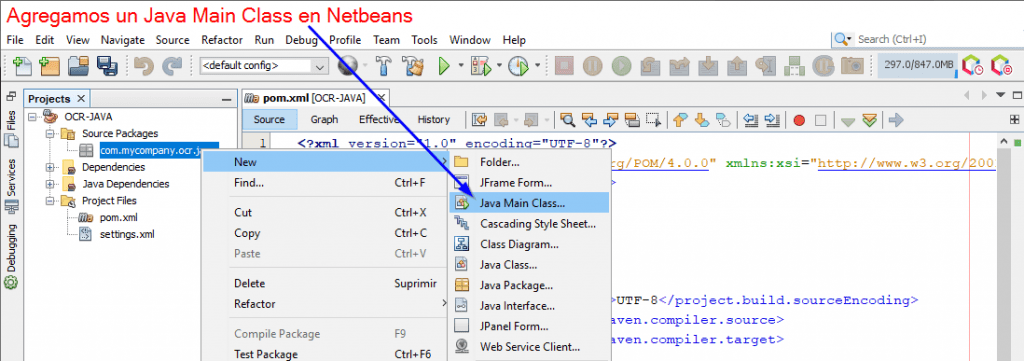
Colocamos un nombre y daos click en finish
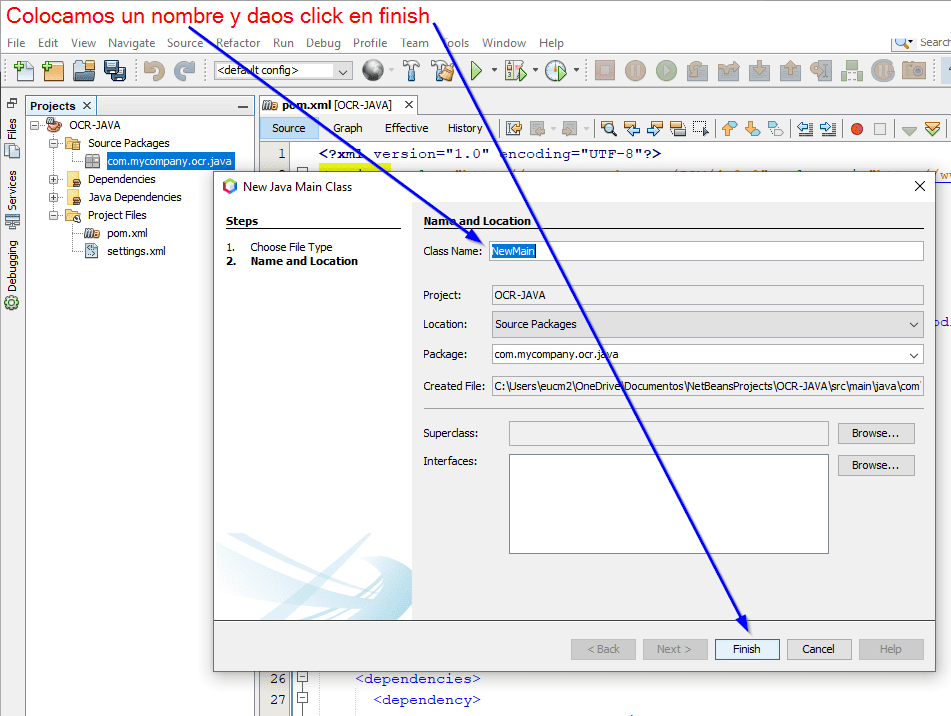
En la raiz del proyecto de Netbeans descargamos la imagen de esta url https://raw.githubusercontent.com/GoogleCloudPlatform/python-docs-samples/main/vision/snippets/quickstart/resources/wakeupcat.jpg
Importamos las dependencias de Google visión:
import com.google.cloud.vision.v1.AnnotateImageRequest; import com.google.cloud.vision.v1.AnnotateImageResponse; import com.google.cloud.vision.v1.BatchAnnotateImagesResponse; import com.google.cloud.vision.v1.EntityAnnotation; import com.google.cloud.vision.v1.Feature; import com.google.cloud.vision.v1.Feature.Type; import com.google.cloud.vision.v1.Image; import com.google.cloud.vision.v1.ImageAnnotatorClient; import com.google.protobuf.ByteString; import java.io.FileInputStream; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.util.ArrayList; import java.util.List; import java.util.logging.Level; import java.util.logging.Logger;
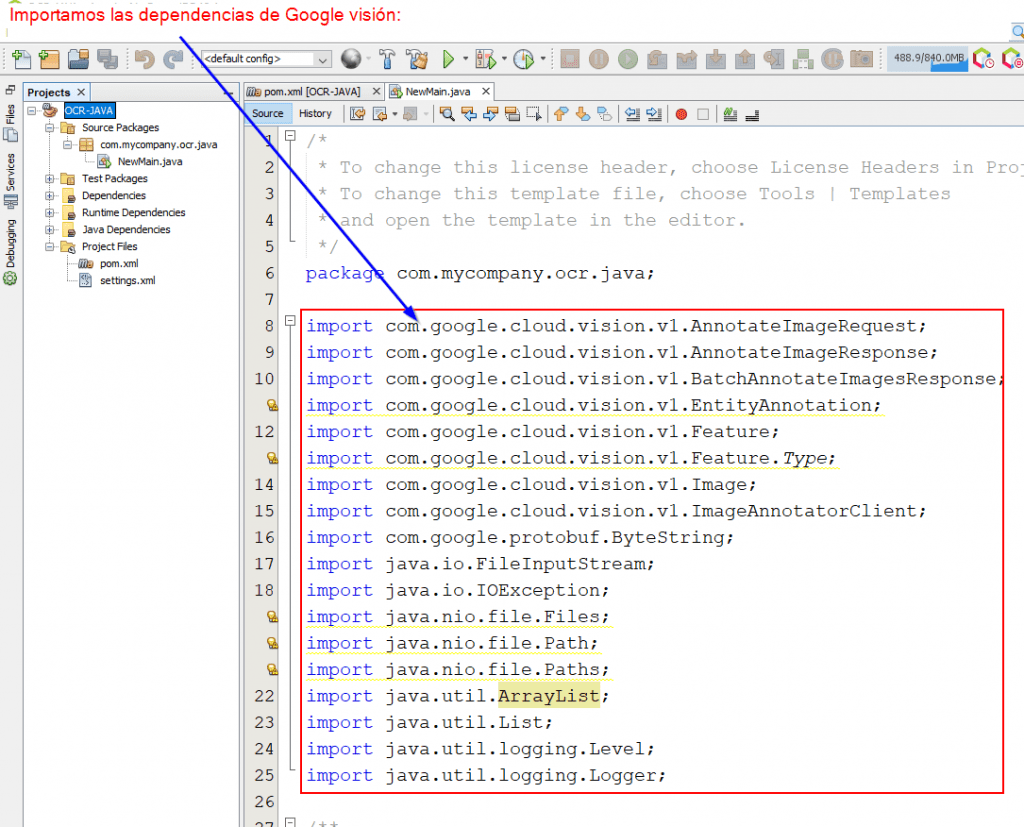
Agregamos la función detectText
public static String detectText(String filePath) throws IOException { List<AnnotateImageRequest> requests = new ArrayList<>(); ByteString imgBytes = ByteString.readFrom(new FileInputStream(filePath)); Image img = Image.newBuilder().setContent(imgBytes).build(); Feature feat = Feature.newBuilder().setType(Feature.Type.TEXT_DETECTION).build(); AnnotateImageRequest request = AnnotateImageRequest.newBuilder().addFeatures(feat).setImage(img).build(); requests.add(request); String texto = ""; // Initialize client that will be used to send requests. This client only needs to be created // once, and can be reused for multiple requests. After completing all of your requests, call // the "close" method on the client to safely clean up any remaining background resources. try (ImageAnnotatorClient client = ImageAnnotatorClient.create()) { BatchAnnotateImagesResponse response = client.batchAnnotateImages(requests); List<AnnotateImageResponse> responses = response.getResponsesList(); for (AnnotateImageResponse res : responses) { if (res.hasError()) { System.out.format("Error: %s%n", res.getError().getMessage()); return null; } // For full list of available annotations, see http://g.co/cloud/vision/docs texto = res.getTextAnnotationsList().get(0).getDescription(); } } return texto; }
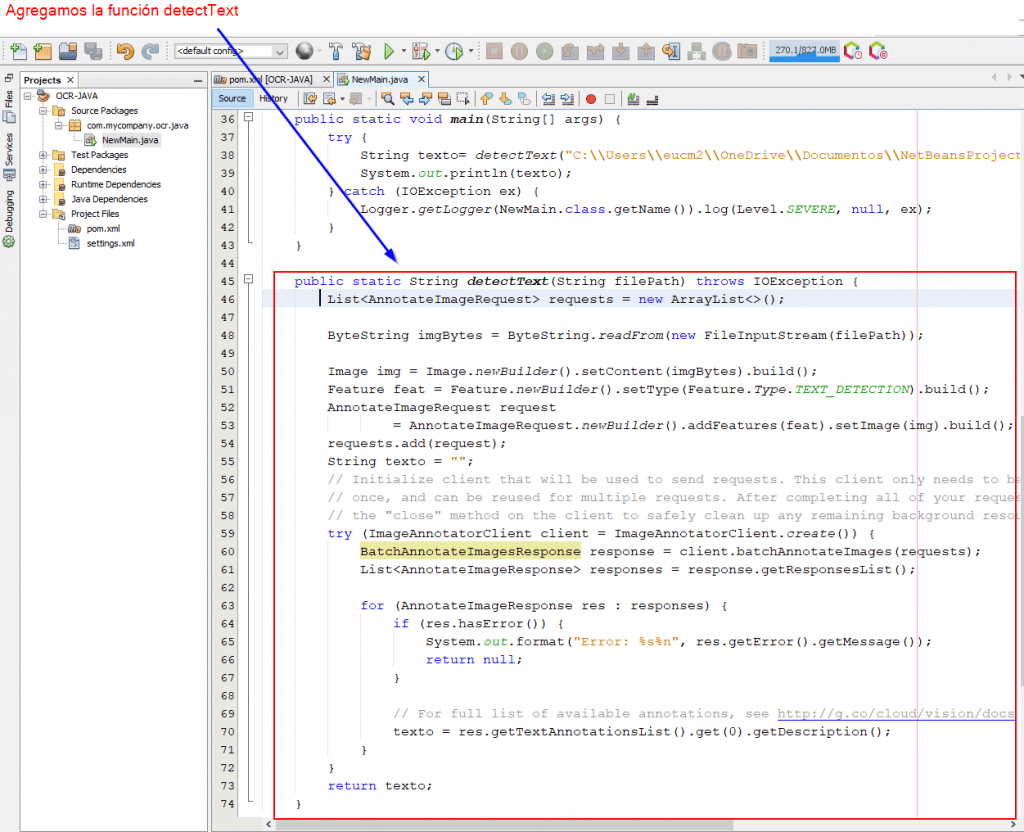
En el método main llamamos al método detectText y mostramos el texto de la imagen
public static void main(String[] args) { try { String texto= detectText("C:\\Users\\eucm2\\OneDrive\\Documentos\\NetBeansProjects\\OCR-JAVA\\wakeupcat.jpg"); System.out.println(texto); } catch (IOException ex) { Logger.getLogger(NewMain.class.getName()).log(Level.SEVERE, null, ex); } }
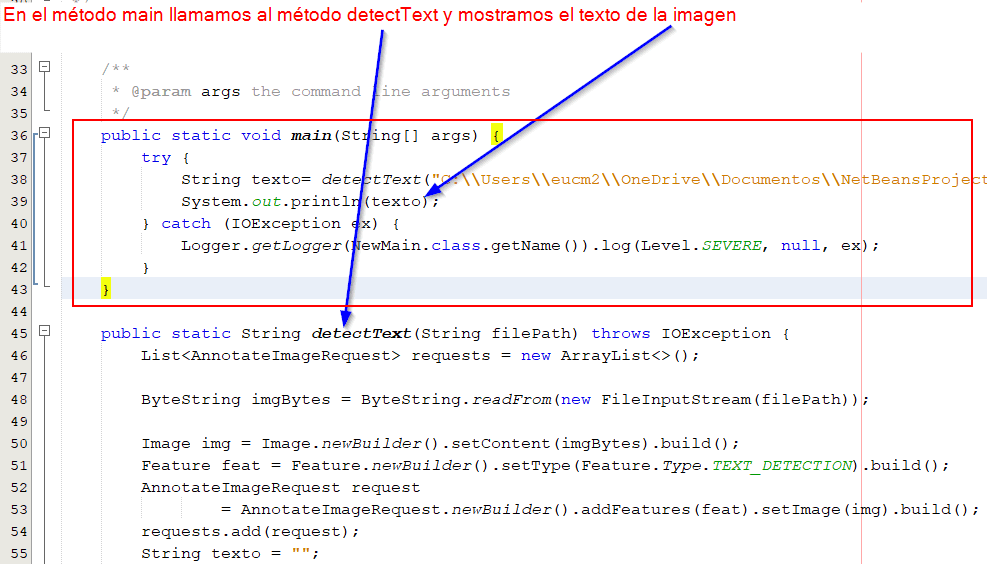
Corremos el proyecto en la consola se nos muestra el texto de la imagen
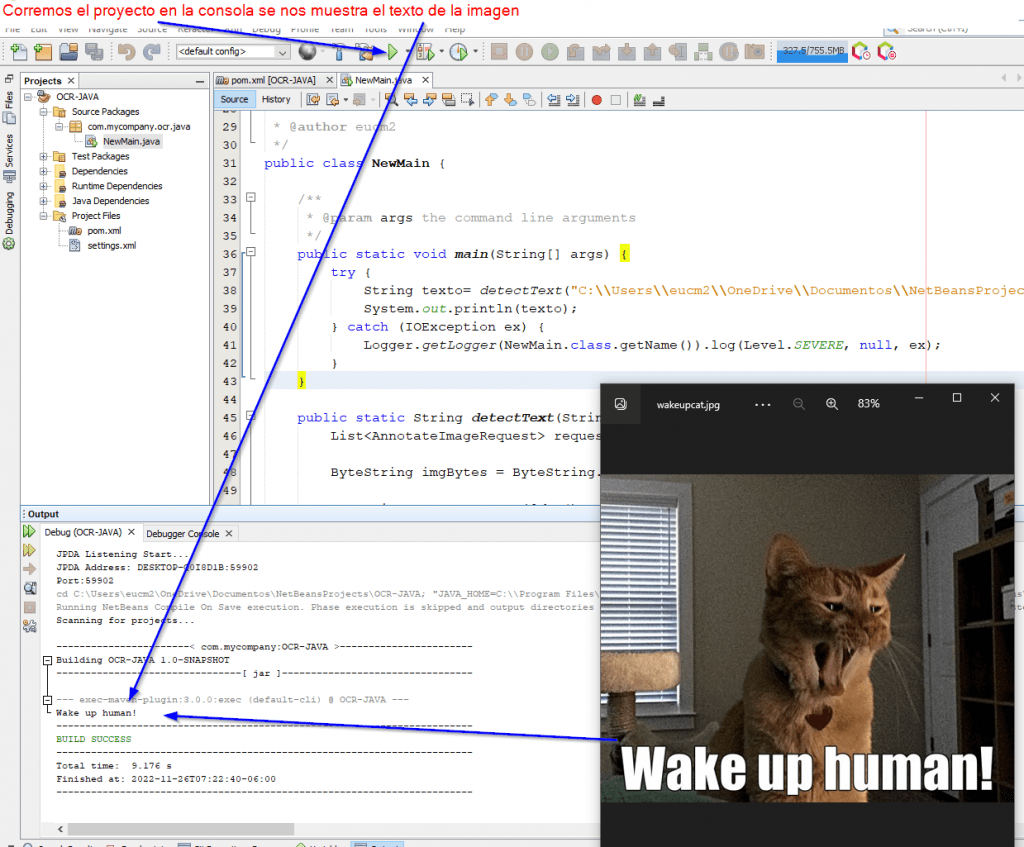
Codigo completo para convertir de imágenes a texto con Java
/* Convertir de imágenes a texto con Java * To change this license header, choose License Headers in Project Properties. * To change this template file, choose Tools | Templates * and open the template in the editor. */ package com.mycompany.ocr.java; import com.google.cloud.vision.v1.AnnotateImageRequest; import com.google.cloud.vision.v1.AnnotateImageResponse; import com.google.cloud.vision.v1.BatchAnnotateImagesResponse; import com.google.cloud.vision.v1.EntityAnnotation; import com.google.cloud.vision.v1.Feature; import com.google.cloud.vision.v1.Feature.Type; import com.google.cloud.vision.v1.Image; import com.google.cloud.vision.v1.ImageAnnotatorClient; import com.google.protobuf.ByteString; import java.io.FileInputStream; import java.io.IOException; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.util.ArrayList; import java.util.List; import java.util.logging.Level; import java.util.logging.Logger; /** * * @author eucm2 */ public class NewMain { /** * @param args the command line arguments */ public static void main(String[] args) { try { String texto= detectText("C:\\Users\\eucm2\\OneDrive\\Documentos\\NetBeansProjects\\OCR-JAVA\\wakeupcat.jpg"); System.out.println(texto); } catch (IOException ex) { Logger.getLogger(NewMain.class.getName()).log(Level.SEVERE, null, ex); } } public static String detectText(String filePath) throws IOException { List<AnnotateImageRequest> requests = new ArrayList<>(); ByteString imgBytes = ByteString.readFrom(new FileInputStream(filePath)); Image img = Image.newBuilder().setContent(imgBytes).build(); Feature feat = Feature.newBuilder().setType(Feature.Type.TEXT_DETECTION).build(); AnnotateImageRequest request = AnnotateImageRequest.newBuilder().addFeatures(feat).setImage(img).build(); requests.add(request); String texto = ""; // Initialize client that will be used to send requests. This client only needs to be created // once, and can be reused for multiple requests. After completing all of your requests, call // the "close" method on the client to safely clean up any remaining background resources. try (ImageAnnotatorClient client = ImageAnnotatorClient.create()) { BatchAnnotateImagesResponse response = client.batchAnnotateImages(requests); List<AnnotateImageResponse> responses = response.getResponsesList(); for (AnnotateImageResponse res : responses) { if (res.hasError()) { System.out.format("Error: %s%n", res.getError().getMessage()); return null; } // For full list of available annotations, see http://g.co/cloud/vision/docs texto = res.getTextAnnotationsList().get(0).getDescription(); } } return texto; } }