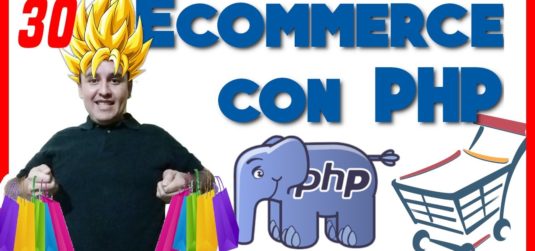
30.-Insertar los registros de detalle de venta en PHP
En este tutorial vamos a insertar los registros de detalle de venta en PHP siguiendo estos pasos:
- 1.- Recibir los datos de nuestro carrito en nuestra factura.
- 2.- Insertar el id venta,id cliente, cantdad, precio y subtotal en nuestr atabla detalle de venta.
- 3.- Insertar múltiples registros con 1 solo query.
Codigo: https://github.com/programadornovato/ecommerce/commit/ac4311a65c5780eb12c1191920beb04d44c9e4d5
admin/js/ecommerce.js
$(document).ready(function () { $.ajax({ type: "post", url: "ajax/leerCarrito.php", dataType: "json", success: function (response) { llenaCarrito(response); } }); $.ajax({ type: "post", url: "ajax/leerCarrito.php", dataType: "json", success: function (response) { llenarTablaCarrito(response); } }); function llenarTablaCarrito(response){ $("#tablaCarrito tbody").text(""); var TOTAL=0; response.forEach(element => { var precio=parseFloat(element['precio']); var totalProd=element['cantidad']*precio; TOTAL=TOTAL+totalProd; $("#tablaCarrito tbody").append( ` <tr> <td><img src="${element['web_path']}" class="img-size-50"/></td> <td>${element['nombre']}</td> <td> ${element['cantidad']} <button type="button" class="btn-xs btn-primary mas" data-id="${element['id']}" data-tipo="mas" >+</button> <button type="button" class="btn-xs btn-danger menos" data-id="${element['id']}" data-tipo="menos" >-</button> </td> <td>$${precio.toFixed(2)}</td> <td>$${totalProd.toFixed(2)}</td> <td><i class="fa fa-trash text-danger borrarProducto" data-id="${element['id']}" ></i></td> <tr> ` ); }); $("#tablaCarrito tbody").append( ` <tr> <td colspan="4" class="text-right"><strong>Total:</strong></td> <td>$${TOTAL.toFixed(2)}</td> <td></td> <tr> ` ); } $.ajax({ type: "post", url: "ajax/leerCarrito.php", dataType: "json", success: function (response) { llenarTablaPasarela(response); } }); function llenarTablaPasarela(response){ $("#tablaPasarela tbody").text(""); var TOTAL=0; response.forEach(element => { var precio=parseFloat(element['precio']); var totalProd=element['cantidad']*precio; TOTAL=TOTAL+totalProd; $("#tablaPasarela tbody").append( ` <tr> <td><img src="${element['web_path']}" class="img-size-50"/></td> <td>${element['nombre']}</td> <td> ${element['cantidad']} <input type="hidden" name="id[]" value="${element['id']}"> <input type="hidden" name="cantidad[]" value="${element['cantidad']}"> <input type="hidden" name="precio[]" value="${precio.toFixed(2)}"> </td> <td>$${precio.toFixed(2)}</td> <td>$${totalProd.toFixed(2)}</td> <tr> ` ); }); $("#tablaPasarela tbody").append( ` <tr> <td colspan="4" class="text-right"><strong>Total:</strong></td> <td> $${TOTAL.toFixed(2)} <input type="hidden" name="total" value="${TOTAL.toFixed(2)*100}" > </td> <tr> ` ); } $(document).on("click",".mas,.menos",function(e){ e.preventDefault(); var id=$(this).data('id'); var tipo=$(this).data('tipo'); $.ajax({ type: "post", url: "ajax/cambiaCantidadProductos.php", data: {"id":id,"tipo":tipo}, dataType: "json", success: function (response) { llenarTablaCarrito(response); llenaCarrito(response); } }); }); $(document).on("click",".borrarProducto",function(e){ e.preventDefault(); var id=$(this).data('id'); $.ajax({ type: "post", url: "ajax/borrarProductoCarrito.php", data: {"id":id}, dataType: "json", success: function (response) { llenarTablaCarrito(response); llenaCarrito(response); } }); }); $("#agregarCarrito").click(function (e) { e.preventDefault(); var id=$(this).data('id'); var nombre=$(this).data('nombre'); var web_path=$(this).data('web_path'); var cantidad=$("#cantidadProducto").val(); var precio=$(this).data('precio'); $.ajax({ type: "post", url: "ajax/agregarCarrito.php", data: {"id":id,"nombre":nombre,"web_path":web_path,"cantidad":cantidad,"precio":precio}, dataType: "json", success: function (response) { llenaCarrito(response); $("#badgeProducto").hide(500).show(500).hide(500).show(500).hide(500).show(500); $("#iconoCarrito").click(); } }); }); function llenaCarrito(response){ var cantidad=Object.keys(response).length; if(cantidad>0){ $("#badgeProducto").text(cantidad); }else{ $("#badgeProducto").text(""); } $("#listaCarrito").text(""); response.forEach(element => { $("#listaCarrito").append( ` <a href="index.php?modulo=detalleproducto&id=${element['id']}" class="dropdown-item"> <!-- Message Start --> <div class="media"> <img src="${element['web_path']}" class="img-size-50 mr-3 img-circle"> <div class="media-body"> <h3 class="dropdown-item-title"> ${element['nombre']} <span class="float-right text-sm text-primary"><i class="fas fa-eye"></i></span> </h3> <p class="text-sm">Cantidad ${element['cantidad']}</p> </div> </div> <!-- Message End --> </a> <div class="dropdown-divider"></div> ` ); }); $("#listaCarrito").append( ` <a href="index.php?modulo=carrito" class="dropdown-item dropdown-footer text-primary"> Ver carrito <i class="fa fa-cart-plus"></i> </a> <div class="dropdown-divider"></div> <a href="#" class="dropdown-item dropdown-footer text-danger" id="borrarCarrito"> Borrar carrito <i class="fa fa-trash"></i> </a> ` ); } $(document).on("click","#borrarCarrito",function(e){ e.preventDefault(); $.ajax({ type: "post", url: "ajax/borrarCarrito.php", dataType: "json", success: function (response) { llenaCarrito(response); } }); }); var nombreRec=$("#nombreRec").val(); var emailRec=$("#emailRec").val(); var direccionRec=$("#direccionRec").val(); $("#jalar").click(function (e) { var nombreCli=$("#nombreCli").val(); var emailCli=$("#emailCli").val(); var direccionCli=$("#direccionCli").val(); if( $(this).prop("checked")==true ){ $("#nombreRec").val(nombreCli); $("#emailRec").val(emailCli); $("#direccionRec").val(direccionCli); }else{ $("#nombreRec").val(nombreRec); $("#emailRec").val(emailRec); $("#direccionRec").val(direccionRec); } }); });
factura.php
<?php $total=$_REQUEST['total']??''; include_once "stripe/init.php"; \Stripe\Stripe::setApiKey("sk_test_JaTXHOFLk3lllnj1PnpahfxR00NLGmUe8M"); $toke=$_POST['stripeToken']; $charge=\Stripe\Charge::create([ 'amount'=>$total, 'currency'=>'usd', 'description'=>'Pago de ecommerce', 'source'=>$toke ]); if($charge['captured']){ $queryVenta="INSERT INTO ventas (idCli ,idPago ,fecha) values ('".$_SESSION['idCliente']."','".$charge['id']."',now()); "; $resVenta=mysqli_query($con,$queryVenta); $id=mysqli_insert_id($con); /* if($resVenta){ echo "La venta fue exitosa con el id=".$id; } */ $insertaDetalle=""; $cantProd=count($_REQUEST['id']); for($i=0;$i<$cantProd;$i++){ $subTotal=$_REQUEST['precio'][$i]*$_REQUEST['cantidad'][$i]; $insertaDetalle=$insertaDetalle."('".$_REQUEST['id'][$i]."','$id','".$_REQUEST['cantidad'][$i]."','".$_REQUEST['precio'][$i]."','$subTotal'),"; } $insertaDetalle=rtrim($insertaDetalle,","); $queryDetalle="INSERT INTO detalleVentas (id, idProd, idVenta, cantidad, precio, subTotal) values $insertaDetalle;"; $resDetalle=mysqli_query($con,$queryDetalle); if($resVenta && $resDetalle){ } } ?>
Cuenta de github de stripe: https://github.com/stripe/stripe-php
Documentacion de stripe: https://stripe.com/docs/stripe-js
Datos de tarjeta de prueba: https://developers.todopago.com.ar/site/datos-de-prueba
🎦Curso de PHP🐘 y MySql🐬: https://www.youtube.com/playlist?list=PLCTD_CpMeEKS2Dvb-WNrAuDAXObB8GzJ0
🎦[Curso] Laravel Tutorial en Español: https://www.youtube.com/playlist?list=PLCTD_CpMeEKQcVcM4u4qddLYRE37S_0XS
🎦Curso]Ajax con Jquery de 0 a 100 🌇: https://www.youtube.com/watch?v=52yI0xiB73A&list=PLCTD_CpMeEKSYJ1J15M8PknOMwOpeqsXz
🎦Mysql configurar una replicación maestro – esclavo 🐬: https://www.youtube.com/watch?v=RY-EdBOJWEs
🎦[Curso] Visual Studio Code 🆚 de 0 a 100: https://www.youtube.com/playlist?list=PLCTD_CpMeEKQbdlT8efsS-veXuvYZ1UWn
🎦[Curso] Bootstrap de 0 a 100 🌈: https://www.youtube.com/playlist?list=PLCTD_CpMeEKSVmsZJIumVvfDviuW-c9AT
🎦[Curso] HTML y CSS de 0 a 100 🌐: https://www.youtube.com/playlist?list=PLCTD_CpMeEKS1SmufBGPOV1mjNfCiLwek
🎦 Esta lista de reproducción: https://www.youtube.com/playlist?list=PLCTD_CpMeEKQhRiJx7Wv3pM3rYvT9_CS9 .
Codigos en gdrive: https://drive.google.com/file/d/1QW8ExkL8eS7nQ5HTDvUuSkkGJMSmecGV/view?usp=sharing
Gracias por apoyar este canal: https://www.patreon.com/programadornovato?fan_landing=true
🔗 Facebook: https://facebook.com/ProgramadorNovatoOficial
🔗 Twitter: https://twitter.com/programadornova
🔗 Linkedin: https://www.linkedin.com/in/programadornovato/
🔗 Instagram: https://www.instagram.com/programadornovato/
<<Anterior tutorial Siguiente tutorial >>