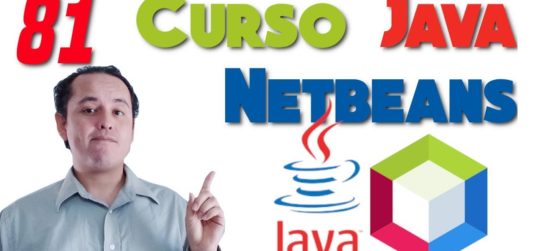
81.- Crear un boton con Java en Netbeans
En este tutorial vamos a crear un crear un boton con Java en Netbeans y ademas jugaremos con algunas de sus propiedades. Por si fuera poco vamos a comparar “AWT Button” con “Swing JButton”.
Java swing: https://es.wikipedia.org/wiki/Swing_(biblioteca_gr%C3%A1fica)
Codigo: https://github.com/programadornovato/java/commit/cb76ba47f8e29e2005b0a1de3b575ebbfdcf4857
package com.programadornovato.proy1; import java.awt.Button; import java.awt.Color; import java.awt.Dimension; import java.awt.Font; import java.awt.HeadlessException; import java.awt.Image; import java.awt.event.KeyEvent; import java.util.ArrayList; import javax.swing.BoxLayout; import javax.swing.ImageIcon; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JLabel; import javax.swing.JOptionPane; import javax.swing.JPanel; import javax.swing.SwingConstants; /** * * @author eugenio */ public class Ventana extends JFrame{ ArrayList <JPanel> panel=new ArrayList<JPanel>(); ArrayList <JLabel> etiqueta=new ArrayList<JLabel>(); JButton b1; Button awtb; int num=4; public Ventana(String title) throws HeadlessException, InterruptedException { super(title); //Dimension d=new Dimension(500, 500); //this.setSize(d); this.setDefaultCloseOperation(EXIT_ON_CLOSE); //this.setLocation(300, 300); this.setBounds(300, 300, 500, 500); this.setLocationRelativeTo(null); //this.num= Integer.parseInt(JOptionPane.showInputDialog("Humano cuantos paneles quieres")); //iniciarPaneles(); //inicaEtiquetas(); iniciarBotones(); } protected void iniciarPaneles() { JPanel contenedor=new JPanel(); this.getContentPane().add(contenedor); contenedor.setBackground(Color.red); for(int i=0;i<this.num;i++){ this.panel.add(new JPanel()); contenedor.add(this.panel.get(i)); this.panel.get(i).setBackground(new Color(i*50, i*50, i*50)); } contenedor.setLayout(new BoxLayout(contenedor, BoxLayout.X_AXIS)); } protected void inicaEtiquetas() { for(int i=0;i<this.num;i++){ this.etiqueta.add(new JLabel("Hola"+(i+1), new ImageIcon(new ImageIcon("images/netbeans 11.png").getImage().getScaledInstance(60, 60, Image.SCALE_DEFAULT)) , SwingConstants.RIGHT )); this.etiqueta.get(i).setForeground(Color.white); this.panel.get(i).add(this.etiqueta.get(i)); } } public void setTextos(String textos[]){ } protected void iniciarBotones() { b1=new JButton("Dale like!!!"); awtb=new Button("Entiende que le des like!!!"); JPanel contenedor=new JPanel(); this.getContentPane().add(contenedor); contenedor.add(b1); contenedor.add(awtb); contenedor.setLayout(null); b1.setBounds(100, 100, 180, 30); awtb.setBounds(100 , 300 , 180, 30); b1.setText("Que le des like!!!"); awtb.setLabel(""); b1.setEnabled(true); b1.setMnemonic(KeyEvent.VK_J); } }
🔗 Instalar Netbeans 11 en Ubuntu con Snap [Mas fácil que en windows?]: https://www.youtube.com/watch?v=LllPPV9SMzQ
🔗 Instalar Netbeans 11 en Windows 10: https://www.youtube.com/watch?v=EouitrKS6Cw
🔗 Descargar e Instalar Netbeans 11 en ubuntu 18 04: https://www.youtube.com/watch?v=tWiX3Z5t5kQ
🔗 Netbeans Sublime Theme ?: https://www.youtube.com/watch?v=oAF2Q7mTZZM
🔗 Editar CSS directamenete en Chrome con Netbeans: https://www.youtube.com/watch?v=HlQs0a7R2cY
🔗 Esta lista de reproducción: https://www.youtube.com/playlist?list=PLCTD_CpMeEKTT-qEHGqZH3fkBgXH4GOTF
Codigos en gdrive: https://drive.google.com/file/d/1M6c0VYqrzpq6KwdWkrkw7Aalm8FkdITH/view?usp=sharing
Gracias por apoyar este canal: https://www.patreon.com/programadornovato?fan_landing=true
🔗 Facebook: https://facebook.com/ProgramadorNovatoOficial
🔗 Twitter: https://twitter.com/programadornova
🔗 Linkedin: https://www.linkedin.com/in/programadornovato/
🔗 Instagram: https://www.instagram.com/programadornovato/
Anterior tutorial Siguiente tutorial