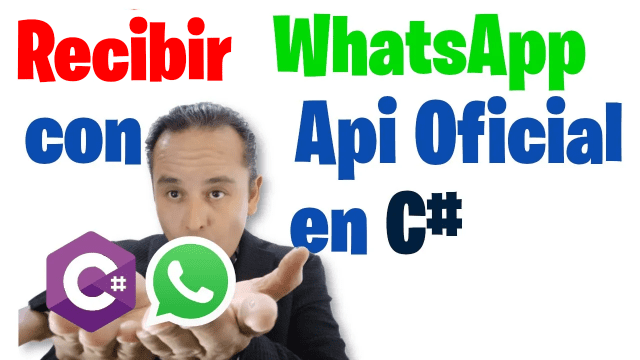
Recibir mensajes con Api Oficial de WhatsApp en C#
En este tutorial aprenderemos a Recibir mensajes con Api Oficial de WhatsApp en C#, solo debemos seguir estos pasos:
Creamos una app en Facebook
O podemos aceder a este link Todas las apps – Meta for Developers (facebook.com)
Configuramos el Api de WhatsApp
Recibir mensajes con Api Oficial de WhatsApp en C#
//ClienteControl.cs using Microsoft.AspNetCore.Mvc; using System.Net; using System.Net.Http.Headers; using WebApiWA.Models; namespace WebApiWA.Controllers { public class ClienteControl:ControllerBase { //RECIBIMOS LOS DATOS DE VALIDACION VIA GET [HttpGet] //DENTRO DE LA RUTA webhook [Route("webhook")] //RECIBIMOS LOS PARAMETROS QUE NOS ENVIA WHATSAPP PARA VALIDAR NUESTRA URL public string Webhook( [FromQuery(Name = "hub.mode")] string mode, [FromQuery(Name = "hub.challenge")] string challenge, [FromQuery(Name = "hub.verify_token")] string verify_token ) { //SI EL TOKEN ES hola (O EL QUE COLOQUEMOS EN FACEBOOK) if (verify_token.Equals("hola")) { return challenge; } else { return ""; } } //RECIBIMOS LOS DATOS DE VIA POST [HttpPost] //DENTRO DE LA RUTA webhook [Route("webhook")] //RECIBIMOS LOS DATOS Y LOS GUARDAMOS EN EL MODELO WebHookResponseModel public dynamic datos([FromBody] WebHookResponseModel entry) { //ESTRAEMOS EL MENSAJE RECIBIDO string mensaje_recibido = entry.entry[0].changes[0].value.messages[0].text.body; //ESTRAEMOS EL ID UNICO DEL MENSAJE string id_wa = entry.entry[0].changes[0].value.messages[0].id; //ESTRAEMOS EL NUMERO DE TELEFONO DEL CUAL RECIBIMOS EL MENSAJE string telefono_wa = entry.entry[0].changes[0].value.messages[0].from; //INICIALIZAMOS LA CONEXION A LA BD Datos dat = new Datos(); //INSERTAMOS LOS DATOS RECIBIDOS dat.insertar(mensaje_recibido, id_wa, telefono_wa); //SI NO HAY ERROR RETORNAMOS UN OK var response = new HttpResponseMessage(HttpStatusCode.OK); response.Content.Headers.ContentType = new MediaTypeHeaderValue("text/plain"); return response; } } }
//Recibir mensajes con Api Oficial de WhatsApp en C# namespace WebApiWA.Models { using MySqlConnector; public class Datos { public void insertar(string mensaje_recibido, string id_wa, string telefono_wa) { var connection = new MySqlConnection("Server=localhost;User ID=root;Password=;Database=aspwa"); try { var command = connection.CreateCommand(); command.CommandText = "INSERT INTO `registro` (`mensaje_recibido`, `id_wa`, `telefono_wa`) VALUES ('" + mensaje_recibido + "', '" + id_wa + "', '" + telefono_wa + "');"; connection.Open(); command.ExecuteNonQuery(); //return "Mitarbeiter wurde angelegt"; } catch (Exception ex) { //return ex.Message; } finally { connection.Close(); } } } public class WebHookResponseModel { public Entry[] entry { get; set; } } public class Entry { public Change[] changes { get; set; } } public class Change { public Value value { get; set; } } public class Value { public int ad_id { get; set; } public long form_id { get; set; } public long leadgen_id { get; set; } public int created_time { get; set; } public long page_id { get; set; } public int adgroup_id { get; set; } public Messages[] messages { get; set; } } public class Messages { public string id { get; set; } public string from { get; set; } public Text text { get; set; } } public class Text { public string body { get; set; } } }
NuGet Gallery | ApiAiSDK 1.6.5
NuGet Gallery | Microsoft.AspNet.WebHooks.Custom 1.2.2
NuGet Gallery | Microsoft.AspNet.WebHooks.Custom.Api 1.2.2
NuGet Gallery | Microsoft.AspNet.WebHooks.Custom.Mvc 1.2.2
NuGet Gallery | MySqlConnector 2.2.5
Creamos la bd en mysql
-- phpMyAdmin SQL Dump -- version 5.1.1 -- https://www.phpmyadmin.net/ -- -- Servidor: 127.0.0.1 -- Tiempo de generación: 03-03-2023 a las 11:32:44 -- Versión del servidor: 10.4.21-MariaDB -- Versión de PHP: 8.0.12 SET SQL_MODE = "NO_AUTO_VALUE_ON_ZERO"; START TRANSACTION; SET time_zone = "+00:00"; /*!40101 SET @OLD_CHARACTER_SET_CLIENT=@@CHARACTER_SET_CLIENT */; /*!40101 SET @OLD_CHARACTER_SET_RESULTS=@@CHARACTER_SET_RESULTS */; /*!40101 SET @OLD_COLLATION_CONNECTION=@@COLLATION_CONNECTION */; /*!40101 SET NAMES utf8mb4 */; -- -- Base de datos: `aspwa` -- CREATE DATABASE IF NOT EXISTS `aspwa` DEFAULT CHARACTER SET utf8mb4 COLLATE utf8mb4_general_ci; USE `aspwa`; -- -------------------------------------------------------- -- -- Estructura de tabla para la tabla `registro` -- CREATE TABLE `registro` ( `id` int(5) NOT NULL, `fecha_hora` datetime DEFAULT current_timestamp(), `mensaje_recibido` varchar(1000) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci DEFAULT '', `id_wa` varchar(1000) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci DEFAULT '', `telefono_wa` varchar(50) CHARACTER SET utf8mb4 COLLATE utf8mb4_unicode_ci DEFAULT '' ) ENGINE=InnoDB DEFAULT CHARSET=utf8mb4; -- -- Índices para tablas volcadas -- -- -- Indices de la tabla `registro` -- ALTER TABLE `registro` ADD PRIMARY KEY (`id`); -- -- AUTO_INCREMENT de las tablas volcadas -- -- -- AUTO_INCREMENT de la tabla `registro` -- ALTER TABLE `registro` MODIFY `id` int(5) NOT NULL AUTO_INCREMENT; COMMIT; /*!40101 SET CHARACTER_SET_CLIENT=@OLD_CHARACTER_SET_CLIENT */; /*!40101 SET CHARACTER_SET_RESULTS=@OLD_CHARACTER_SET_RESULTS */; /*!40101 SET COLLATION_CONNECTION=@OLD_COLLATION_CONNECTION */;
Ave que vuela, a la cazuela.